Totaling
Check out this worksheet:
Here's code for the button:
total
is initialized to zero. Then the loop starts. The CPU runs line 6 again and again. The first time, row
is 1. Then 2, and so on, up to 5. Line 6 grabs Cells(row, 1)
and adds it to total
. The first time through the loop, row
is 1, so Cells(1, 1)
is added to total
. The next time through the loop, Cells(2, 1)
is added, and so on, up to Cells(5, 1)
.
total
is an accumulator. It's a variable that tracks something about a data set during a loop.
Accumulators should always be initialized. In this program, that's in line 4:
total
is added to each time through the loop, with a different cell.
At the end of the loop, total
describes something about the entire data set.
Don't rely on the value of the index variable (row
in the example) after a For-Next loop. It's predictable in some cases, but not in others.
Totals that aren't
In the example, total
is the simple sum of the cells:
total = total + Cells(row, 1)
But it doesn't have to be. For example, in statistics, you sometimes want the sum of squares. That is, take each value, square it, and add those up. It's used in, for example, calculating standard deviations.
This line would compute the sum of squares:
sumSq = sumSq + Cells(row, 1) * Cells(row, 1)
You can do both calculations at once:
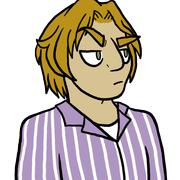
total
and sum
. I know they mean the same thing, but would it be better to be consistent?
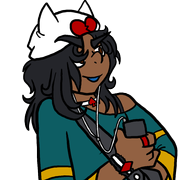
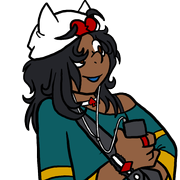
Highest
Accumulators aren't just for totals. You can use them for all sorts of things, like finding the highest value. Here's that worksheet again:
Here's the code:
highest
is an accumulator. As the loop runs, it holds the highest value that the program has found so far. At the end of the loop, it holds the highest value in the entire data set.
The initialization is different:
Rather than initializing to 0, the program grabs the first data value for highest
. Then it loops through the rest of the data, comparing each element with highest
.
Cell with the highest
Suppose that we didn't want to know the highest number, but the cell that contained the highest number. So for this…
… it's not 92 we want, it's 4. That's the row with the highest number in it.
Easy peasy.
Instead of using an accumulator to track the highest value, we use an accumulator to track the cell with the highest value.
Lowest
Almost the same as the highest code. Let's add to the code we have.
Exercises
Upload your workbook.
(If you were logged in as a student, you could submit an exercise solution, and get some feedback.)
The number of data items is given in cell B7.
Add code that computes the average, highest value, and lowest value of the data set. Make sure the program works when the amount of data changes.
Validate n, the number of data items. If the user enters a value less than 2, show this:
Upload your file.
(If you were logged in as a student, you could submit an exercise solution, and get some feedback.)
Summary
An accumulator is a variable that tracks something about a data set during a loop. Accumulators should always be initialized.